Understanding API Fundamentals
APIs (Application Programming Interfaces) enable communication between software systems, forming the foundation of interconnected applications. Their design must account for scalability, security, and maintainability to meet increasing demands and evolving requirements. Robust APIs improve developer experience, system adaptability, and performance under load.
Requirements and Endpoint Design
Stakeholder and Requirement Analysis
Before implementation, engage stakeholders to gather both functional requirements (e.g., user login, data submission) and non-functional requirements (e.g., latency thresholds, availability, security). Understanding these early helps prevent misalignment and supports a user-centered design process.
RESTful Endpoint Design
API endpoints are the gateway to functionality. Each should be tied to a specific resource and follow RESTful conventions:
- Use nouns in URIs to represent resources (e.g.,
/users
instead of/getUser
) - Apply standard HTTP methods like
GET
,POST
,PUT
, andDELETE
- Keep routes intuitive and organized
A consistent naming convention and clear structure make it easier for developers to consume and integrate your API. Additionally, anticipate future extensibility by designing endpoints that won’t break existing clients when enhanced.
Data Handling: Formats, Versioning & Efficiency
Choosing the right data format—JSON for readability or Protobuf for speed—impacts performance and integration. Proper versioning (via URL paths, query parameters, or hybrid approaches) maintains backward compatibility as APIs evolve. Implementing rate limiting, throttling, and efficient request handling ensures APIs remain responsive during high traffic.
Security and Access Control
Authentication and Authorization
Distinguish between authentication (verifying identity) and authorization (granting access). Common mechanisms:
- OAuth 2.0: Ideal for delegated access (e.g., social logins)
- JWTs: Stateless and efficient for validating sessions
- API keys: Simple, but best paired with IP restrictions and role scopes
Always use TLS/mTLS to encrypt communications and protect credentials in transit.
Regular Security Maintenance
Keep your APIs hardened with regular patching and vulnerability scans. APIs are high-value attack surfaces, and staying updated is key to protecting sensitive data. Develop a routine for monitoring, auditing, and applying security fixes proactively.
Architecture and Data Optimization
Database Strategies
Choose the right data store based on your API’s access patterns:
- SQL for relational, structured queries and transactional integrity
- NoSQL for scalable, high-throughput, flexible-schema applications
Optimization techniques include:
- Indexing for faster reads
- Sharding to split large datasets across instances
- Replication for fault tolerance and read scaling
Microservices Architecture
Splitting your application into microservices allows teams to manage, deploy, and scale components independently. This increases development velocity, fault isolation, and technology flexibility. Services can be optimized individually for performance and infrastructure use.
Performance Optimization: Caching, Load Balancing & Async
Caching strategies (server-side, CDN, client-side) reduce redundant processing and speed up response times. Load balancing techniques—Round Robin, Least Connections, IP Hashing—distribute requests evenly, avoiding bottlenecks. Asynchronous processing with message queues and event-driven workflows improves scalability and responsiveness for high-load environments.
Deployment, Monitoring, and Logging
CI/CD Pipelines
Automate the development lifecycle with CI/CD tools like Jenkins, GitHub Actions, or CircleCI. Benefits include:
- Faster releases
- Fewer deployment errors
- Better test coverage and rollback readiness
Incorporate automated security testing to catch vulnerabilities early.
Observability
Use logging and monitoring tools to maintain API health:
- Prometheus: Metrics and time-series tracking
- Grafana: Dashboards for real-time analysis
- ELK Stack: Centralized log collection and visualization
Effective observability enables fast incident response and long-term performance tuning.
Developer Experience and Documentation
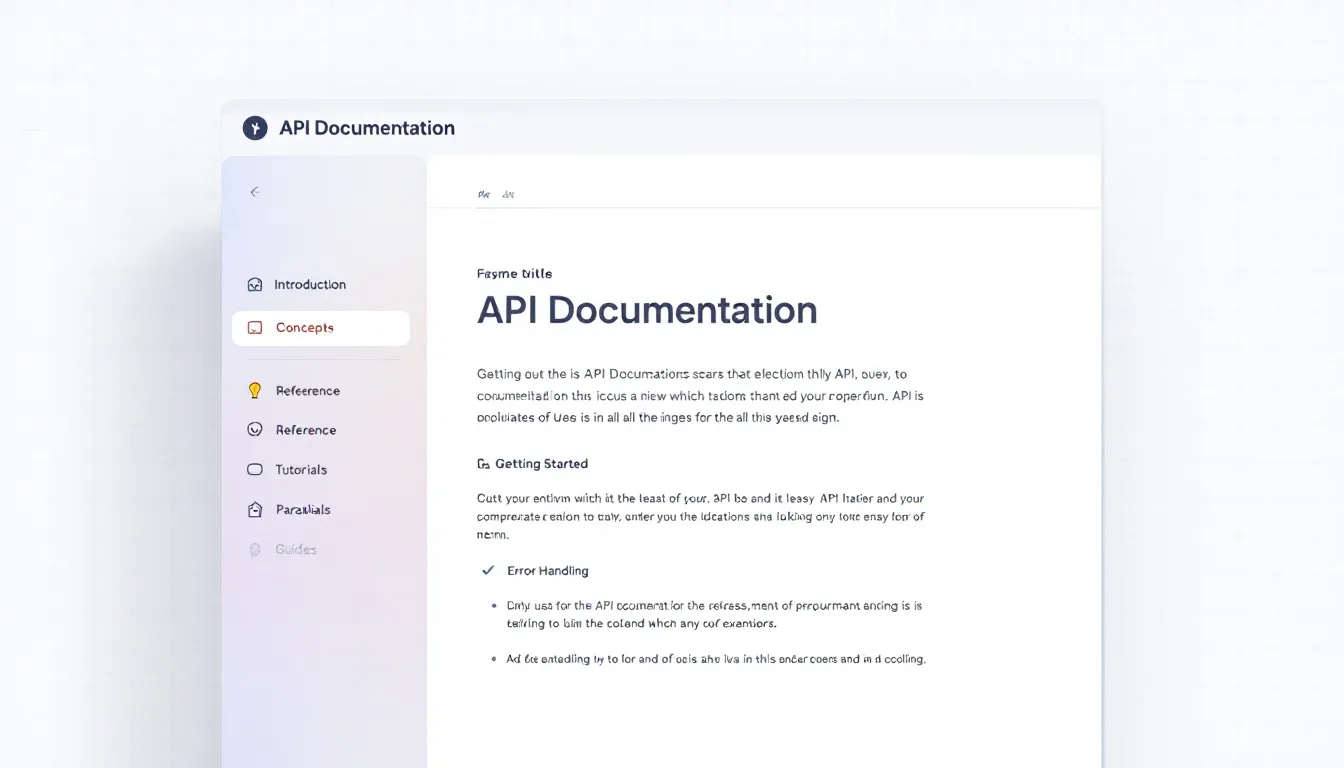
High-quality documentation reduces onboarding time and boosts adoption. Include:
- Clear endpoint definitions
- Request/response samples
- Authentication setup
- Error handling guides
Use tools like Swagger, Postman, and OpenAPI for interactive documentation. Offering mock endpoints and live testing further enhances the developer experience. Always gather feedback from developers to improve usability.
Scaling Strategies
To meet growing demand, APIs can scale vertically (more resources per server) or horizontally (more servers). Horizontal scaling offers better flexibility, especially when paired with auto-scaling policies and effective monitoring. A dynamic approach ensures consistent performance during traffic spikes and minimizes downtime.
Summary
Secure and scalable API development requires more than writing working code—it demands strategic design, efficient architecture, robust security, continuous testing, and a great developer experience. From endpoint design to load balancing and CI/CD, every layer contributes to a resilient API that scales with demand.
Following these best practices ensures your APIs are well-architected, secure, and ready to support evolving business needs—making them a dependable backbone of any modern application.